400
|
How can I display the column using currency format and enlarge the font for certain values
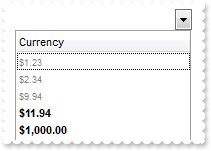
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Currency")));
var_Column->PutDef(EXCOMBOBOXLib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"len(value) ? ((0:=dbl(value)) < 10 ? '<fgcolor=808080><font ;7>' : '<b>') + currency(=:0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("9.94");
var_Items->AddItem("11.94");
var_Items->AddItem("1000");
|
399
|
How can I get the number of occurrences of a specified string in the cell
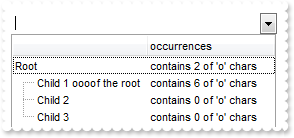
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"occurrences")));
var_Column->PutComputedField(L"lower(%0) count 'o'");
var_Column->PutFormatColumn(L"'contains ' + value + ' of \\'o\\' chars'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1 oooof the root");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
398
|
How can I display dates in my format
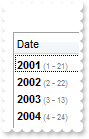
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Date")));
var_Column->PutDef(EXCOMBOBOXLib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"'<b>' + year(0:=date(value)) + '</b><fgcolor=808080><font ;6> (' + month(=:0) + ' - ' + day(=:0) +')'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,21,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,24,0,00,00).operator DATE());
|
397
|
How can I display dates in short format
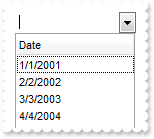
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Date")))->PutFormatColumn(L"shortdate(value)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,0,00,00).operator DATE());
|
396
|
How can I display dates in long format
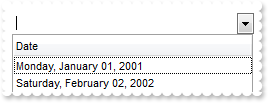
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Date")))->PutFormatColumn(L"longdate(value)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,0,00,00).operator DATE());
|
395
|
How can I display only the right part of the cell
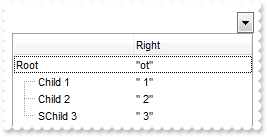
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Right")));
var_Column->PutComputedField(L"%0 right 2");
var_Column->PutFormatColumn(L"'\"' + value + '\"'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"SChild 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
394
|
How can I display only the left part of the cell
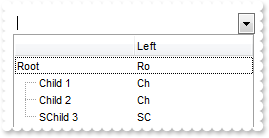
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Left")))->PutComputedField(L"%0 left 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"SChild 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
393
|
How can I display true or false instead 0 and -1
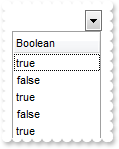
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Boolean")))->PutFormatColumn(L"value != 0 ? 'true' : 'false'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(VARIANT_TRUE);
var_Items->AddItem(VARIANT_FALSE);
var_Items->AddItem(VARIANT_TRUE);
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
|
392
|
How can I display icons or images instead numbers
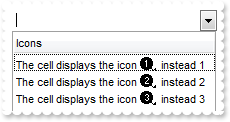
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Icons")));
var_Column->PutDef(EXCOMBOBOXLib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"'The cell displays the icon <img>'+value+'</img> instead ' + value");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
var_Items->AddItem(long(3));
|
391
|
How can I display the column using currency

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Currency")))->PutFormatColumn(L"currency(dbl(value))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("0");
var_Items->AddItem(long(5));
var_Items->AddItem("10000.99");
|
390
|
How can I display the currency only for not empty cells
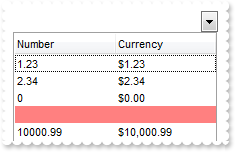
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Currency")))->PutComputedField(L"len(%0) ? currency(dbl(%0)) : ''");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("0");
var_Items->PutItemBackColor(var_Items->AddItem(vtMissing),RGB(255,128,128));
var_Items->AddItem("10000.99");
|
389
|
Is there a function to display the number of days between two date including the number of hours
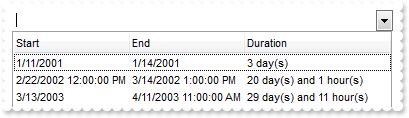
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Start")))->PutWidth(32);
spComboBox1->GetColumns()->Add(L"End");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Duration")))->PutComputedField(_bstr_t("2:=((1:=int(0:= date(%1)-date(%0))) = 0 ? '' : str(=:1) + ' day(s)') + ( 3:=round(24*(=:0-floor(=:0))) ? (len(=:2) ? ' and ' : ") +
"'') + =:3 + ' hour(s)' : '' )");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,12,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,13,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,11,00,00).operator DATE());
|
388
|
Is there a function to display the number of days between two date including the number of hours
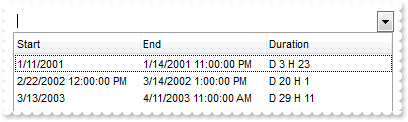
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Start");
spComboBox1->GetColumns()->Add(L"End");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Duration")))->PutComputedField(L"\"D \" + int(date(%1)-date(%0)) + \" H \" + round(24*(date(%1)-date(%0) - floor(date(%1)-date(%0))))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,23,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,12,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,13,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,11,00,00).operator DATE());
|
387
|
How can I display the number of days between two dates
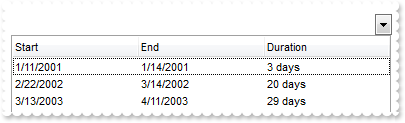
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Start");
spComboBox1->GetColumns()->Add(L"End");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Duration")))->PutComputedField(L"(date(%1)-date(%0)) + ' days'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,0,00,00).operator DATE());
|
386
|
How can I get second part of the date
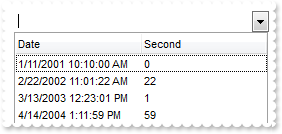
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Second")))->PutComputedField(L"sec(date(%0))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,10,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,01,22).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,23,01).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,11,59).operator DATE());
|
385
|
How can I get minute part of the date
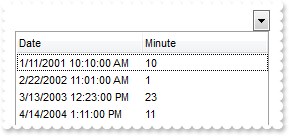
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Minute")))->PutComputedField(L"min(date(%0))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,10,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,01,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,23,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,11,00).operator DATE());
|
384
|
How can I check the hour part only so I know it was afternoon
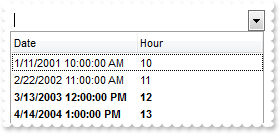
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"hour(%0)>=12",vtMissing)->PutBold(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Hour")))->PutComputedField(L"hour(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
383
|
What about a function to get the day in the week, or days since Sunday
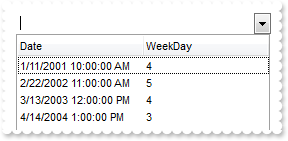
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"WeekDay")))->PutComputedField(L"weekday(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
382
|
Is there any function to get the day of the year or number of days since January 1st
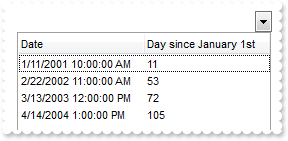
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Day since January 1st")))->PutComputedField(L"yearday(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
381
|
How can I display only the day of the date
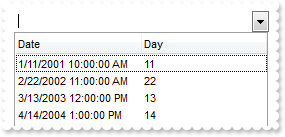
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Day")))->PutComputedField(L"day(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
380
|
How can I display only the month of the date
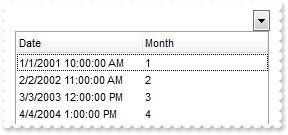
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Month")))->PutComputedField(L"month(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,13,00,00).operator DATE());
|
379
|
How can I get only the year part from a date expression
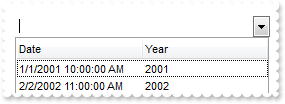
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Year")))->PutComputedField(L"year(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,13,00,00).operator DATE());
|
378
|
Can I convert the expression to date
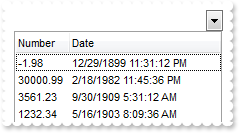
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Date")))->PutComputedField(L"date(dbl(%0))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("30000.99");
var_Items->AddItem("3561.23");
var_Items->AddItem("1232.34");
|
377
|
Can I convert the expression to a number, double or float
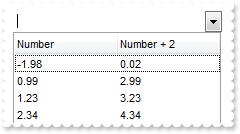
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Number + 2")))->PutComputedField(L"dbl(%0)+2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
376
|
How can I display dates in long format
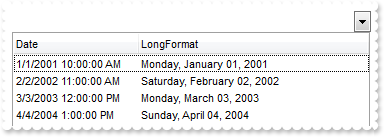
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"LongFormat")))->PutComputedField(L"longdate(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,13,00,00).operator DATE());
|
375
|
How can I display dates in short format
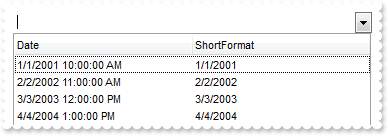
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"ShortFormat")))->PutComputedField(L"shortdate(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,13,00,00).operator DATE());
|
374
|
How can I display the time only of a date expression
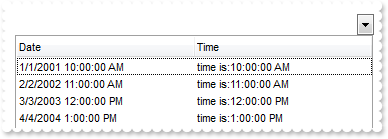
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Date");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Time")))->PutComputedField(L"'time is:' + time(date(%0))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,13,00,00).operator DATE());
|
373
|
Is there any function to display currencies, or money formatted as in the control panel
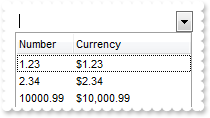
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Currency")))->PutComputedField(L"currency(dbl(%0))");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("10000.99");
|
372
|
How can I convert the expression to a string so I can look into the date string expression for month's name
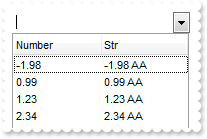
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Str")))->PutComputedField(L"str(%0) + ' AA'");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
371
|
Can I display the absolute value or positive part of the number
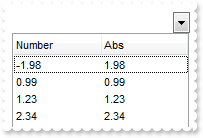
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Abs")))->PutComputedField(L"abs(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
370
|
Is there any function to get largest number with no fraction part that is not greater than the value
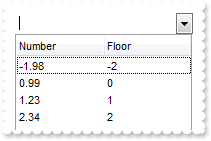
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Floor")))->PutComputedField(L"floor(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
369
|
Is there any function to round the values base on the .5 value
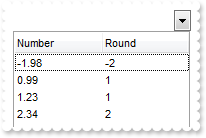
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Round")))->PutComputedField(L"round(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
368
|
How can I get or display the integer part of the cell
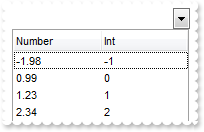
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Number");
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Int")))->PutComputedField(L"int(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("-1.98");
var_Items->AddItem("0.99");
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
|
367
|
How can I display names as proper ( first leter of the word must be in uppercase, and the rest in lowercase )
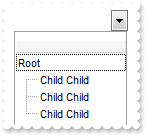
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"")))->PutComputedField(L"proper(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("root");
var_Items->InsertItem(h,vtMissing,"child child");
var_Items->InsertItem(h,vtMissing,"child child");
var_Items->InsertItem(h,vtMissing,"child child");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
366
|
Is there any option to display cells in uppercase
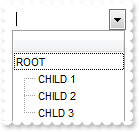
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"")))->PutComputedField(L"upper(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Chld 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
365
|
Is there any option to display cells in lowercase
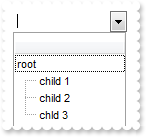
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"")))->PutComputedField(L"lower(%0)");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Chld 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
364
|
How can I mark the cells that has a specified type, ie strings only
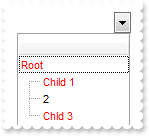
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"type(%0) = 8",vtMissing)->PutForeColor(RGB(255,0,0));
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,long(2));
var_Items->InsertItem(h,vtMissing,"Chld 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
363
|
How can I bold the items that contains data or those who displays empty strings
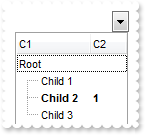
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"not len(%1)=0",vtMissing)->PutBold(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
long hC = var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutCellCaption(hC,long(1),"1");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
362
|
Can I change the background color for items or cells that contains a specified string
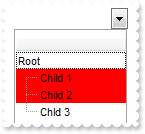
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"%0 contains 'hi'",vtMissing)->PutBackColor(RGB(255,0,0));
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Chld 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
361
|
Is there any option to change the fore color for cells or items that ends with a specified string
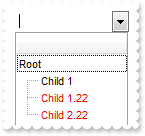
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"%0 endwith '22'",vtMissing)->PutForeColor(RGB(255,0,0));
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 1.22");
var_Items->InsertItem(h,vtMissing,"Child 2.22");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
360
|
How can I highlight the cells or items that starts with a specified string
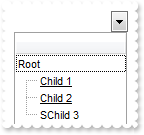
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetConditionalFormats()->Add(L"%0 startwith 'C'",vtMissing)->PutUnderline(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"SChild 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
359
|
How can I change the background color or the visual appearance using ebn for a particular column
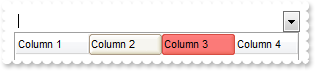
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
EXCOMBOBOXLib::IColumnsPtr var_Columns = spComboBox1->GetColumns();
var_Columns->Add(L"Column 1");
((EXCOMBOBOXLib::IColumnPtr)(var_Columns->Add(L"Column 2")))->PutDef(EXCOMBOBOXLib::exHeaderBackColor,long(16777216));
((EXCOMBOBOXLib::IColumnPtr)(var_Columns->Add(L"Column 3")))->PutDef(EXCOMBOBOXLib::exHeaderBackColor,long(16777471));
var_Columns->Add(L"Column 4");
|
358
|
How can I change the background color for a particular column
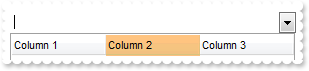
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
EXCOMBOBOXLib::IColumnsPtr var_Columns = spComboBox1->GetColumns();
var_Columns->Add(L"Column 1");
((EXCOMBOBOXLib::IColumnPtr)(var_Columns->Add(L"Column 2")))->PutDef(EXCOMBOBOXLib::exHeaderBackColor,long(8439039));
var_Columns->Add(L"Column 3");
|
357
|
Does your control support prompt feature
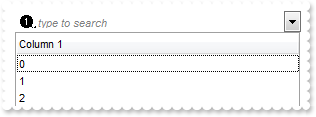
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spComboBox1->PutAutoComplete(VARIANT_FALSE);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Column 1")))->PutPrompt(L"<img>1</img><i><fgcolor=808080>type to search</fgcolor></i>");
spComboBox1->GetItems()->AddItem(long(0));
spComboBox1->GetItems()->AddItem(long(1));
spComboBox1->GetItems()->AddItem(long(2));
|
356
|
How can I display the column's header using multiple lines
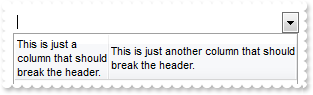
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutHeaderHeight(128);
spComboBox1->PutHeaderSingleLine(VARIANT_FALSE);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"This is just a column that should break the header.")))->PutWidth(32);
spComboBox1->GetColumns()->Add(L"This is just another column that should break the header.");
|
355
|
How can I sort the value gets listed in the drop down filter window
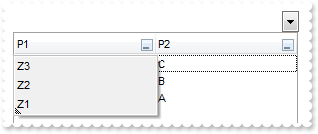
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exLinesAtRoot);
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutDescription(EXCOMBOBOXLib::exFilterBarAll,L"");
spComboBox1->PutDescription(EXCOMBOBOXLib::exFilterBarBlanks,L"");
spComboBox1->PutDescription(EXCOMBOBOXLib::exFilterBarNonBlanks,L"");
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"P1")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXCOMBOBOXLib::exSortItemsDesc);
EXCOMBOBOXLib::IColumnPtr var_Column1 = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"P2")));
var_Column1->PutDisplayFilterButton(VARIANT_TRUE);
var_Column1->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column1->PutFilterList(EXCOMBOBOXLib::exSortItemsAsc);
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Z3");
var_Items->PutCellCaption(h,long(1),"C");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Z1"),long(1),"B");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Z2"),long(1),"A");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
354
|
Is there any property to disable the popup/context menu being shown when the user does a right click in the control's label area
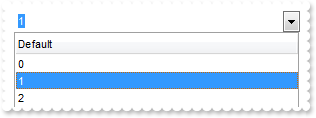
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Default")))->PutAllowEditContextMenu(VARIANT_FALSE);
spComboBox1->GetItems()->AddItem(long(0));
spComboBox1->GetItems()->AddItem(long(1));
spComboBox1->GetItems()->AddItem(long(2));
|
353
|
How can I align the text/caption on the scroll bar
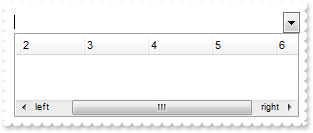
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutScrollPartCaption(EXCOMBOBOXLib::exHScroll,EXCOMBOBOXLib::exLowerBackPart,L"left");
spComboBox1->PutScrollPartCaptionAlignment(EXCOMBOBOXLib::exHScroll,EXCOMBOBOXLib::exLowerBackPart,EXCOMBOBOXLib::LeftAlignment);
spComboBox1->PutScrollPartCaption(EXCOMBOBOXLib::exHScroll,EXCOMBOBOXLib::exUpperBackPart,L"right");
spComboBox1->PutScrollPartCaptionAlignment(EXCOMBOBOXLib::exHScroll,EXCOMBOBOXLib::exUpperBackPart,EXCOMBOBOXLib::RightAlignment);
spComboBox1->PutColumnAutoResize(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"1");
spComboBox1->GetColumns()->Add(L"2");
spComboBox1->GetColumns()->Add(L"3");
spComboBox1->GetColumns()->Add(L"4");
spComboBox1->GetColumns()->Add(L"5");
spComboBox1->GetColumns()->Add(L"6");
|
352
|
Does you control support RTL languages or if there is a property RightToLeft
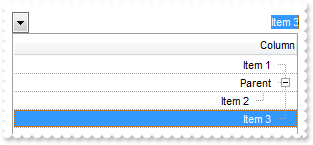
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutRightToLeft(VARIANT_TRUE);
spComboBox1->PutItemsAllowSizing(EXCOMBOBOXLib::exResizeItem);
spComboBox1->PutDrawGridLines(EXCOMBOBOXLib::exHLines);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exLinesAtRoot);
spComboBox1->PutScrollBySingleLine(VARIANT_TRUE);
spComboBox1->PutDefaultItemHeight(64);
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Column")));
var_Column->PutAlignment(EXCOMBOBOXLib::RightAlignment);
var_Column->PutHeaderAlignment(EXCOMBOBOXLib::RightAlignment);
var_Column->PutEditAlignment(EXCOMBOBOXLib::RightAlignment);
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 1");
var_Items->PutItemHeight(var_Items->InsertItem(var_Items->AddItem("Parent"),vtMissing,"Item 2"),48);
var_Items->AddItem("Item 3");
var_Items->PutExpandItem(var_Items->GetItemByIndex(1),VARIANT_TRUE);
|
351
|
How do I enable resizing all the items at runtime
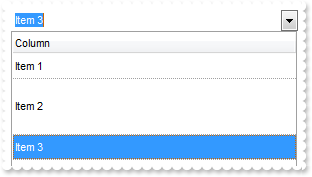
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutItemsAllowSizing(EXCOMBOBOXLib::exResizeAllItems);
spComboBox1->PutDrawGridLines(EXCOMBOBOXLib::exHLines);
spComboBox1->GetColumns()->Add(L"Column");
spComboBox1->GetItems()->AddItem("Item 1");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutItemHeight(var_Items->AddItem("Item 2"),48);
spComboBox1->GetItems()->AddItem("Item 3");
|
350
|
How can I remove the filter

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Column")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutFilterType(EXCOMBOBOXLib::exBlanks);
spComboBox1->ApplyFilter();
spComboBox1->ClearFilter();
|
349
|
How do I display the icons being selected in the control's label
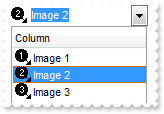
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellImage(var_Items->AddItem("Image 1"),long(0),1);
var_Items->PutCellImage(var_Items->AddItem("Image 2"),long(0),2);
var_Items->PutCellImage(var_Items->AddItem("Image 3"),long(0),3);
spComboBox1->PutAssignEditImageOnSelect(0,VARIANT_TRUE);
spComboBox1->PutValue("Image 2");
|
348
|
How do I select a value
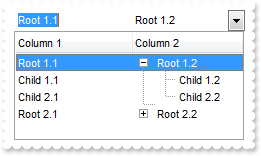
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
spComboBox1->PutValue("Root 1.1");
|
347
|
How do I select a value
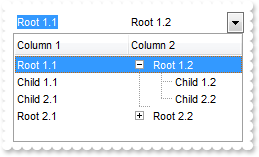
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
spComboBox1->PutSelect(long(1),"Root 1.2");
|
346
|
How do change the visual appearance for the drop down border, using EBN
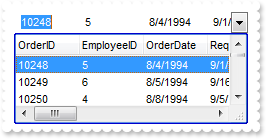
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spComboBox1->PutDropDownBorder(EXCOMBOBOXLib::AppearanceEnum(0x1000000));
|
345
|
How do I remove the drop down's border
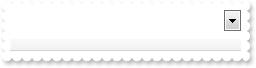
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutDropDownBorder(EXCOMBOBOXLib::None2);
|
344
|
How can I change the foreground color for edit controls
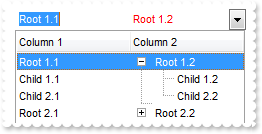
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutForeColorEdit(RGB(255,0,0));
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
spComboBox1->PutSelect(long(0),"Root 1.1");
|
343
|
How can I change the background color for edit controls
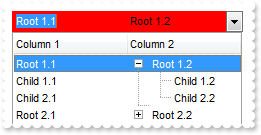
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutBackColorEdit(RGB(255,0,0));
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
spComboBox1->PutSelect(long(0),"Root 1.1");
|
342
|
How can I hide the drop down buttons when the control loses the focus
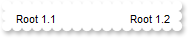
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutHideDropDownButton(VARIANT_TRUE);
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
|
341
|
How can I ensure that the drop down portions doesn't show partial items
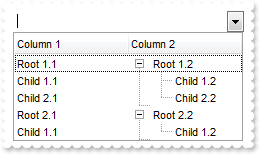
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutIntegralHeight(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
|
340
|
How can I close the drop down window when user double clicks it
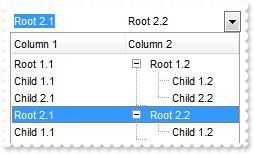
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutCloseOnDblClk(VARIANT_TRUE);
spComboBox1->PutLinesAtRoot(EXCOMBOBOXLib::exGroupLinesAtRoot);
spComboBox1->PutTreeColumnIndex(1);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Root 1.1");
var_Items->PutCellCaption(h,long(1),"Root 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 2.1"),long(1),"Child 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2.1");
var_Items->PutCellCaption(h,long(1),"Root 2.2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Child 1.1"),long(1),"Child 1.2");
|
339
|
How do I get the handle of the drop down window

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(_bstr_t(spComboBox1->GethWndDropDown()));
|
338
|
How do I specify the height of the control's label

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutLabelHeight(34);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
337
|
The control selects the portion of text that doesn't match with the selected item. How can I avoid that
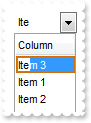
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAutoSelect(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
336
|
How can I show the drop down window as soon as user starts typing in the control
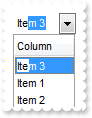
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAutoDropDown(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
335
|
How do I change the text in the edit or label area
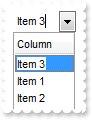
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
spComboBox1->PutEditText(long(0),L"Test");
|
334
|
How do I lock or make read-only the control
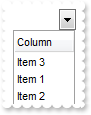
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutLocked(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
333
|
How do I let user to resize only the height of the drop down window, at runtime
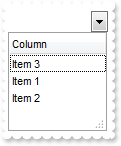
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->PutAllowHResize(VARIANT_FALSE);
spComboBox1->PutMinWidthList(100);
spComboBox1->PutMinHeightList(100);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
332
|
How do I let user to resize only the width of the drop down window, at runtime
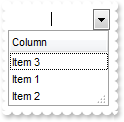
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->PutAllowVResize(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
331
|
How do I let user to resize the drop down window, at runtime
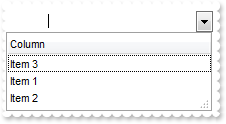
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
330
|
How do I specify the height of the drop down window
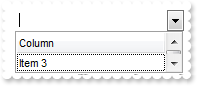
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutHeightList(vtMissing,400);
spComboBox1->PutMinWidthList(100);
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
329
|
How do I specify the minimum height of the drop down window
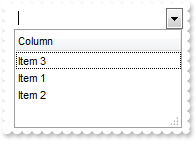
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMinHeightList(100);
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
328
|
How do I specify the width of the drop down window
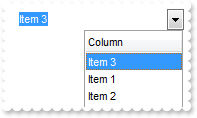
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutWidthList(vtMissing,100);
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
327
|
How do I specify the minimum width of the drop down window
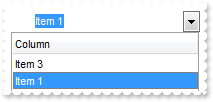
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMinWidthList(100);
spComboBox1->PutAllowSizeGrip(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
326
|
I have multiple columns, how can I display a single edit in the control's label
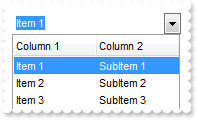
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutSingleEdit(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"Column 1");
spComboBox1->GetColumns()->Add(L"Column 2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("Item 1"),long(1),"SubItem 1");
var_Items->PutCellCaption(var_Items->AddItem("Item 2"),long(1),"SubItem 2");
var_Items->PutCellCaption(var_Items->AddItem("Item 3"),long(1),"SubItem 3");
|
325
|
How do I turn off the auto complete feature
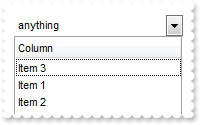
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutAutoComplete(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"Column");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
324
|
The control supports three styles: Simple, DropDown and DropDownList. How can I change the style
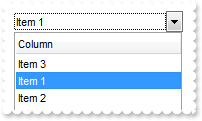
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutStyle(EXCOMBOBOXLib::DropDownList);
|
323
|
Is there any option to align the header to the left and the data to the right
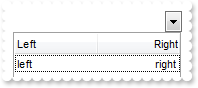
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Left")))->PutAlignment(EXCOMBOBOXLib::LeftAlignment);
EXCOMBOBOXLib::IColumnPtr var_Column = ((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Right")));
var_Column->PutAlignment(EXCOMBOBOXLib::RightAlignment);
var_Column->PutHeaderAlignment(EXCOMBOBOXLib::RightAlignment);
var_Column->PutEditAlignment(EXCOMBOBOXLib::RightAlignment);
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("left"),long(1),"right");
|
322
|
How do I change the control's border, using your EBN files
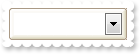
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spComboBox1->PutAppearance(EXCOMBOBOXLib::AppearanceEnum(0x1000000));
|
321
|
Can I change the default border of the tooltip, using your EBN files
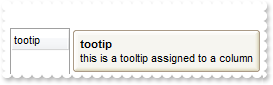
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutToolTipDelay(1);
spComboBox1->PutToolTipWidth(364);
spComboBox1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spComboBox1->PutBackground(EXCOMBOBOXLib::exToolTipAppearance,0x1000000);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"tootip")))->PutToolTip(L"this is a tooltip assigned to a column");
|
320
|
Can I change the background color for the tooltip
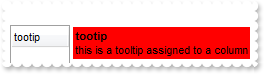
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutToolTipDelay(1);
spComboBox1->PutToolTipWidth(364);
spComboBox1->PutBackground(EXCOMBOBOXLib::exToolTipBackColor,RGB(255,0,0));
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"tootip")))->PutToolTip(L"this is a tooltip assigned to a column");
|
319
|
Does the tooltip support HTML format
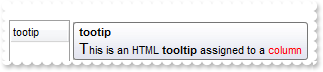
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutToolTipDelay(1);
spComboBox1->PutToolTipWidth(364);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"tootip")))->PutToolTip(L"<font Tahoma;11>T</font>his is an HTML <b>tooltip</b> assigned to a <fgcolor=FF0000>column</fgcolor>");
|
318
|
Can I change the forecolor for the tooltip
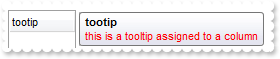
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutToolTipDelay(1);
spComboBox1->PutToolTipWidth(364);
spComboBox1->PutBackground(EXCOMBOBOXLib::exToolTipForeColor,RGB(255,0,0));
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"tootip")))->PutToolTip(L"this is a tooltip assigned to a column");
|
317
|
Can I change the foreground color for the tooltip
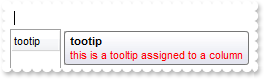
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutToolTipDelay(1);
spComboBox1->PutToolTipWidth(364);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"tootip")))->PutToolTip(L"<fgcolor=FF0000>this is a tooltip assigned to a column</fgcolor>");
|
316
|
How can I merge cells
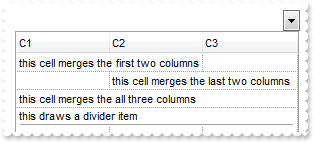
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutDrawGridLines(EXCOMBOBOXLib::exAllLines);
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
spComboBox1->GetColumns()->Add(L"C3");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("this cell merges the first two columns");
var_Items->PutCellMerge(h,long(0),long(1));
h = var_Items->AddItem(vtMissing);
var_Items->PutCellCaption(h,long(1),"this cell merges the last two columns");
var_Items->PutCellMerge(h,long(1),long(2));
h = var_Items->AddItem("this cell merges the all three columns");
var_Items->PutCellMerge(h,long(0),long(1));
var_Items->PutCellMerge(h,long(0),long(2));
h = var_Items->AddItem("this draws a divider item");
var_Items->PutItemDivider(h,0);
|
315
|
How can I merge cells
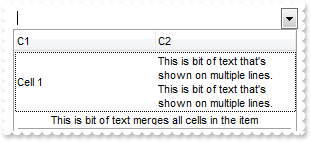
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem("This is bit of text merges all cells in the item");
var_Items->PutItemDivider(h,0);
var_Items->PutCellHAlignment(h,long(0),EXCOMBOBOXLib::CenterAlignment);
|
314
|
How can I change the color for separator / dividers items
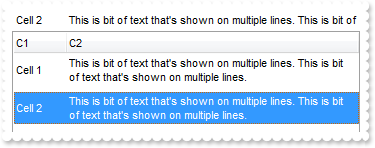
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->PutScrollBySingleLine(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem(vtMissing);
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLine(h,EXCOMBOBOXLib::DoubleDotLine);
var_Items->PutItemDividerLineAlignment(h,EXCOMBOBOXLib::DividerCenter);
var_Items->PutItemHeight(h,6);
var_Items->PutSelectableItem(h,VARIANT_FALSE);
h = var_Items->AddItem("Cell 2");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
|
313
|
How can I add separator - dividers items
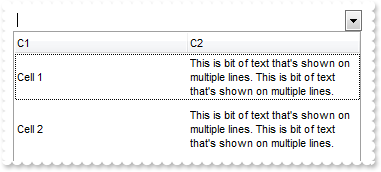
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->PutScrollBySingleLine(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem(vtMissing);
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLine(h,EXCOMBOBOXLib::DoubleDotLine);
var_Items->PutItemDividerLineAlignment(h,EXCOMBOBOXLib::DividerCenter);
var_Items->PutItemHeight(h,6);
var_Items->PutSelectableItem(h,VARIANT_FALSE);
h = var_Items->AddItem("Cell 2");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
|
312
|
Can I change the style of the line being displayed by a divider item
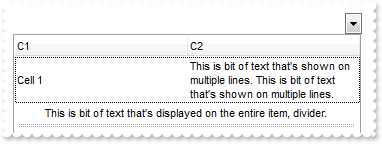
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->PutScrollBySingleLine(VARIANT_FALSE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem("This is bit of text that's displayed on the entire item, divider.");
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLine(h,EXCOMBOBOXLib::DoubleDotLine);
var_Items->PutCellHAlignment(h,long(0),EXCOMBOBOXLib::CenterAlignment);
var_Items->PutItemHeight(h,24);
|
311
|
Can I remove the line being displayed by a divider item
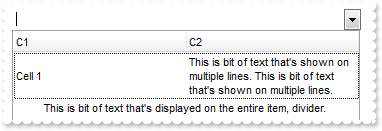
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem("This is bit of text that's displayed on the entire item, divider.");
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLine(h,EXCOMBOBOXLib::EmptyLine);
var_Items->PutCellHAlignment(h,long(0),EXCOMBOBOXLib::CenterAlignment);
|
310
|
How can I display a divider item, merging all cells
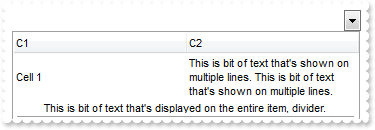
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutMarkSearchColumn(VARIANT_FALSE);
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
h = var_Items->AddItem("This is bit of text that's displayed on the entire item, divider.");
var_Items->PutItemDivider(h,0);
var_Items->PutCellHAlignment(h,long(0),EXCOMBOBOXLib::CenterAlignment);
|
309
|
How can I fix or lock items
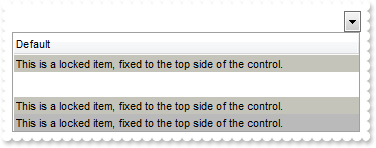
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Default");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutLockedItemCount(EXCOMBOBOXLib::exTop,1);
var_Items->PutCellCaption(var_Items->GetLockedItem(EXCOMBOBOXLib::exTop,0),long(0),"This is a locked item, fixed to the top side of the control.");
var_Items->PutItemBackColor(var_Items->GetLockedItem(EXCOMBOBOXLib::exTop,0),RGB(196,196,186));
var_Items->PutLockedItemCount(EXCOMBOBOXLib::exBottom,2);
var_Items->PutCellCaption(var_Items->GetLockedItem(EXCOMBOBOXLib::exBottom,0),long(0),"This is a locked item, fixed to the top side of the control.");
var_Items->PutItemBackColor(var_Items->GetLockedItem(EXCOMBOBOXLib::exBottom,0),RGB(196,196,186));
var_Items->PutCellCaption(var_Items->GetLockedItem(EXCOMBOBOXLib::exBottom,1),long(0),"This is a locked item, fixed to the top side of the control.");
var_Items->PutItemBackColor(var_Items->GetLockedItem(EXCOMBOBOXLib::exBottom,1),RGB(186,186,186));
|
308
|
How can I fix or lock an item on the bottom side of the control
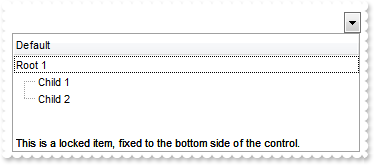
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Default");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutLockedItemCount(EXCOMBOBOXLib::exBottom,1);
var_Items->PutCellCaption(var_Items->GetLockedItem(EXCOMBOBOXLib::exBottom,0),long(0),"This is a locked item, fixed to the bottom side of the control.");
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
307
|
How can I fix or lock an item on the top of the control
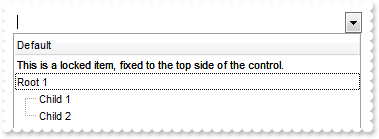
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Default");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutLockedItemCount(EXCOMBOBOXLib::exTop,1);
var_Items->PutCellCaption(var_Items->GetLockedItem(EXCOMBOBOXLib::exTop,0),long(0),"This is a locked item, fixed to the top side of the control.");
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
306
|
Is there any function to limit the height of the items when I display it using multiple lines
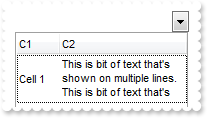
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutScrollBySingleLine(VARIANT_TRUE);
spComboBox1->GetColumns()->Add(L"C1");
spComboBox1->GetColumns()->Add(L"C2");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"This is bit of text that's shown on multiple lines. This is bit of text that's shown on multiple lines.");
var_Items->PutCellSingleLine(h,long(1),EXCOMBOBOXLib::exCaptionWordWrap);
var_Items->PutItemMaxHeight(h,48);
|
305
|
Why I cannot center my cells in the column
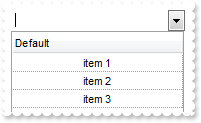
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->PutDrawGridLines(EXCOMBOBOXLib::exRowLines);
((EXCOMBOBOXLib::IColumnPtr)(spComboBox1->GetColumns()->Add(L"Default")))->PutAlignment(EXCOMBOBOXLib::CenterAlignment);
spComboBox1->GetItems()->AddItem("item 1");
spComboBox1->GetItems()->AddItem("item 2");
spComboBox1->GetItems()->AddItem("item 3");
|
304
|
How can I align the cell to the left, center or to the right
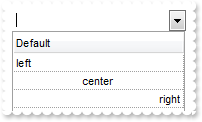
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->PutDrawGridLines(EXCOMBOBOXLib::exRowLines);
spComboBox1->GetColumns()->Add(L"Default");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellHAlignment(var_Items->AddItem("left"),long(0),EXCOMBOBOXLib::LeftAlignment);
var_Items->PutCellHAlignment(var_Items->AddItem("center"),long(0),EXCOMBOBOXLib::CenterAlignment);
var_Items->PutCellHAlignment(var_Items->AddItem("right"),long(0),EXCOMBOBOXLib::RightAlignment);
|
303
|
How do I apply HTML format to a cell
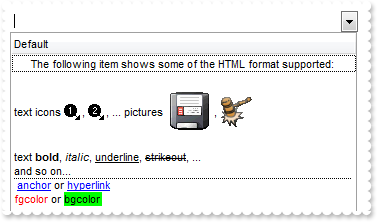
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->PutTreeColumnIndex(-1);
spComboBox1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
spComboBox1->PutHTMLPicture(L"p1","c:\\exontrol\\images\\zipdisk.gif");
spComboBox1->PutHTMLPicture(L"p2","c:\\exontrol\\images\\auction.gif");
spComboBox1->GetColumns()->Add(L"Default");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
long h = var_Items->AddItem("The following item shows some of the HTML format supported:");
var_Items->PutCellHAlignment(h,long(0),EXCOMBOBOXLib::CenterAlignment);
h = var_Items->AddItem(_bstr_t("<br>text icons <img>1</img>, <img>2</img>, ... pictures <img>p1</img>, <img>p2</img> <br><br>text <b>bold</b>, <i>italic</i>, <") +
"u>underline</u>, <s>strikeout</s>, ...<br><dotline>and so on...<br> <a>anchor</a> or <a2>hyperlink</a><br><fgcolor=FF0000>fgcolo" +
"r</fgcolor> or <bgcolor=00FF00>bgcolor</bgcolor> ");
var_Items->PutCellCaptionFormat(h,long(0),EXCOMBOBOXLib::exHTML);
var_Items->PutCellSingleLine(h,long(0),EXCOMBOBOXLib::exCaptionWordWrap);
|
302
|
How can I change the font for a cell
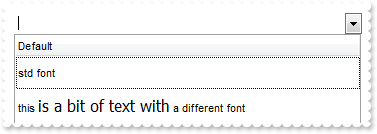
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Default");
spComboBox1->GetItems()->AddItem("std font");
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("this <font tahoma;12>is a bit of text with</font> a different font"),long(0),EXCOMBOBOXLib::exHTML);
|
301
|
How can I change the font for a cell
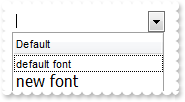
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr spComboBox1 = GetDlgItem(IDC_COMBOBOX1)->GetControlUnknown();
spComboBox1->GetColumns()->Add(L"Default");
spComboBox1->GetItems()->AddItem("default font");
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'stdole' for the library: 'OLE Automation'
#import <stdole2.tlb>
*/
stdole::FontPtr f = ::CreateObject(L"StdFont");
f->PutName(L"Tahoma");
f->PutSize(_variant_t(long(12)));
EXCOMBOBOXLib::IItemsPtr var_Items = spComboBox1->GetItems();
var_Items->PutCellFont(var_Items->AddItem("new font"),long(0),IFontDispPtr(((stdole::FontPtr)(f))));
|